Table of Contents
Getting started with Python is an exciting step toward entering the world of programming. Python’s simplicity and versatility make it a top choice for beginners and professionals alike. However, selecting the right programming language for a project can significantly impact development speed, efficiency, and outcomes. This post will walk you through some of the most popular programming languages and their use cases. We will then focus on setting up Python on your computer, writing your first program, and understanding the basics of Python’s syntax.
What is Programming Language?
A programming language is a formal set of instructions that allows humans to communicate with computers and create software applications. It provides a structured way to write code that can be understood and executed by a computer’s hardware or software. Programming languages follow specific syntax and rules, enabling developers to build websites, applications, algorithms, and more. They come in different types, such as high-level languages like Python and Java, which are easy to learn and widely used, and low-level languages like C and Assembly, which offer greater control over hardware. The choice of programming language depends on the task at hand, as each language has unique features and strengths suited for specific use cases.
Difference Between Compilers and Interpreters
A compiler and an interpreter are tools used to translate high-level programming code into machine-readable instructions that a computer can execute. A compiler converts the entire code at once into machine code, creating a separate executable file. This process makes compiled programs, like those written in C++ or Java, run faster but requires more time upfront for compilation. On the other hand, an interpreter translates and runs code line by line, which allows for easier debugging and quick testing but may result in slower execution. Python is an example of an interpreted language, making it ideal for beginners due to its interactive nature. Both tools play essential roles in programming, with compilers focusing on performance and interpreters prioritizing flexibility.
Let’s see popular programming languages and their use cases:
Programming Language | Overview | Use Cases |
Python | A high-level, versatile language known for its easy-to-read syntax. It is often recommended for beginners. | Data science, web development, AI, automation, machine learning. |
JavaScript | Powers interactive websites and supports both client-side and server-side development via Node.js. | Web development, single-page applications (SPAs) using React, Vue.js, and Angular. |
Java | Known for portability, thanks to the Java Virtual Machine (JVM). | Android apps, enterprise software, large-scale systems. |
C++ | An extension of C with advanced features for low-level programming. | Operating systems, games, real-time simulations, embedded systems. |
C# | Designed by Apple for developing iOS and macOS apps. | iOS and macOS apps, with performance close to C++ but simpler syntax. |
Choosing the right programming language depends on the nature of your project. For beginners, getting started with Python is often the best choice because of its easy-to-understand syntax and widespread use across multiple domains.
Getting Started with Python on Your Computer
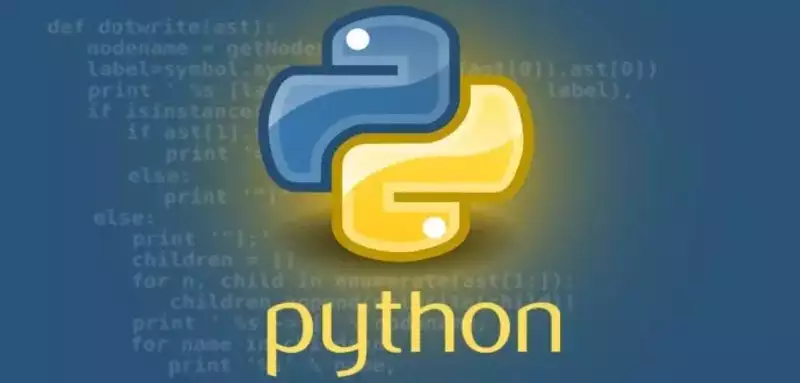
Python uses an interpreter, meaning code is executed line by line, which simplifies debugging. This makes Python an accessible first language, ideal for those just getting started with programming.
Steps to Install and Set Up Python
- Download Python: Visit python.org and download the latest version for your operating system. This is the first step towards getting started with Python.
- Install Python: Follow the installation instructions and check the option to add Python to PATH.
- Verify Installation: Open your command prompt or terminal and type: python –version, If the installation was successful, the Python version will appear.
Steps to Set Up Development Environment
What is Development Environment?
A development environment is a set of tools and software that programmers use to write, test, and debug their code efficiently. It typically includes a text editor or an Integrated Development Environment (IDE), compilers or interpreters, version control systems, and debugging tools. Popular development environments for Python include VS Code, PyCharm, and Jupyter Notebook. These environments streamline the coding process by offering features like syntax highlighting, code suggestions, and error tracking, making development faster and more organized.
You can write Python code using a text editor or an Integrated Development Environment (IDE). Here are some recommended tools:
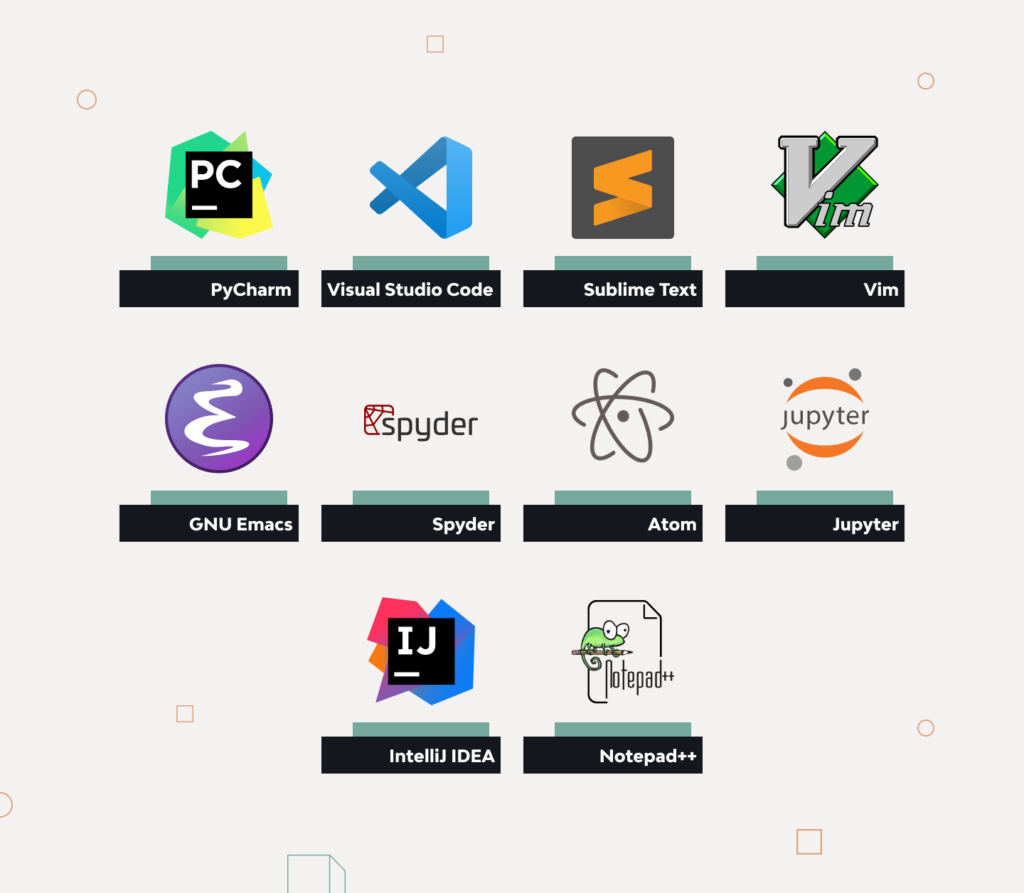
- PyCharm: Best for larger projects. Download from JetBrains.
- VS Code: A lightweight editor with Python support. Download from Microsoft.
- Jupyter Notebook: Ideal for data science. Install it with: pip install notebook
Steps to Write and Run First Python Program
- Open Your Editor or IDE, If you’re using Jupyter Notebook, open a new notebook.
- Write the Code: Type the following:
print("Hello, World!")
- Save Your Program: Save the file as
hello_world.py
. In Jupyter, it saves automatically. - Run the Program: If using Jupyter, run the code cell otherwise In the terminal, navigate to the directory and type:
python hello_world.py
- View the Output: You should see “Hello, World!” displayed on the screen. Congratulations, you’re now officially getting started with Python!
Comments in Python
Comments in Python are lines of text within a program that are ignored by the interpreter, serving as notes to explain the code. They help improve code readability, making it easier for developers to understand the logic or purpose behind certain sections. Comments are also useful when debugging or collaborating with others. In Python, a single-line comment starts with the #
symbol, while multi-line comments can be enclosed within triple quotes ('''
or """
). Though Python doesn’t execute comments, they are essential for writing clean, maintainable code by documenting functionality and intentions. Here’s an example:
# This is a single-line comment
name = "Alice" # Assigning a value to the variable 'name'
"""
This is a multi-line comment
It explains that the code below
prints a greeting message.
"""
print(f"Hello, {name}!")
Using meaningful comments helps both the original developer and others who may review or modify the code later. Now that we have covered everything in Getting started with Python, we will start leaning more about Python syntax in next article!
Conclusion
Getting started with Python is a smooth entry point into programming, thanks to its simplicity and versatility. Python’s syntax is beginner-friendly, and its applications span from web development to data science and AI. Whether you are writing your first program or setting up your development environment, Python offers tools that make learning easy and effective. As you explore other languages like JavaScript, C++, or Java, the knowledge you gain with Python will serve as a solid foundation for your programming journey.
Why is Python a good choice for beginners?
Python is easy to read and learn, with simple syntax. It’s widely used across multiple fields, making it practical for new programmers.
How is an interpreter different from a compiler?
An interpreter executes code line by line, making it easier to debug, while a compiler translates the entire code at once before execution.
What are some popular IDEs for Python?
PyCharm, VS Code, and Jupyter Notebook are popular choices, offering different features based on your needs.
How do I verify if Python is installed correctly?
Open your command prompt or terminal and type python --version
. If Python is installed, the version number will appear.
Can I use Python for web development?
Yes, Python is widely used in web development with frameworks like Django and Flask.
What is the first step to getting started with Python?
Download and install Python from the official website, and set up an IDE or text editor for writing your code.
Is Python suitable for data science?
Absolutely! Python is one of the most popular languages in data science, with libraries like Pandas, NumPy, and SciPy.