Table of Contents
In the world of computer science, data structures and algorithms are the building blocks of efficient programming and problem-solving. They are the secret behind everything from the smooth functioning of search engines to real-time GPS navigation and social media feeds. Mastering data structures and algorithms is essential for developers, data analysts, and anyone aspiring to solve complex computational problems. This post will provide an overview of these fundamental concepts and their real-world applications, helping you lay a solid foundation in programming.
What Are Data Structures and Algorithms?
Data structures refer to specific ways of organizing and storing data so that operations like retrieval, insertion, and deletion can be performed efficiently. On the other hand, algorithms are step-by-step instructions that define how to process and transform data. Combining data structures and algorithms enables you to create powerful solutions that maximize efficiency and minimize resource usage.
Common Types of Data Structures and Their Uses
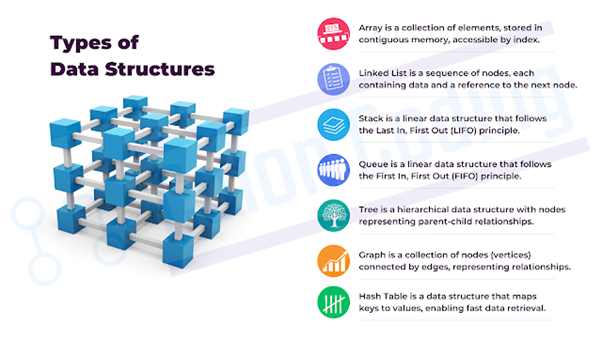
Choosing the right data structure is key to solving a problem effectively. Let’s take a look at some widely used data structures:
Data Structure | Overview | Example Applications |
Arrays | Store elements in contiguous memory locations, accessible via indices. | Used in implementing matrices, storing game boards, and creating lookup tables. |
Linked Lists | A series of connected nodes, where each node points to the next. | Suitable for building playlists, task queues, and managing memory allocation. |
Stacks | A LIFO (Last In, First Out) structure. | Commonly used for undo mechanisms and managing function calls. |
Queues | A FIFO (First In, First Out) structure. | Ideal for scheduling processes, managing requests in servers, and implementing messaging services. |
Trees | A hierarchical structure with parent-child relationships. | Essential in creating directory structures, decision trees, and organizing hierarchical data. |
Graphs | Represent interconnected objects using nodes and edges. | Used in social networks, network routing, and pathfinding algorithms. |
Hash Tables | Store key-value pairs with unique keys mapped to specific values. | Implement dictionaries, caching mechanisms, and database indexing. |
Introduction to Algorithms and Their Importance
Algorithms define the steps needed to manipulate data and solve problems effectively. The performance of an algorithm is measured by its time complexity (speed) and space complexity (memory usage). By learning different types of algorithms, you can approach problems with a variety of strategies and choose the most efficient one. Here are some major types of algorithms:
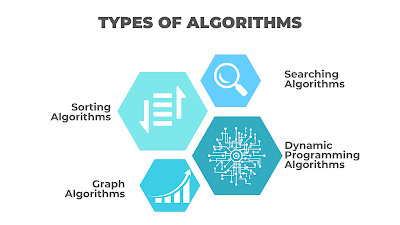
Algorithm Type | Description | Example Use Cases |
Sorting Algorithms | Organize data into a specified order (e.g., Bubble Sort, Quick Sort, Merge Sort). | Used for sorting e-commerce product listings, arranging student grades, and formatting reports. |
Search Algorithms | Locate a specific item within a data structure (e.g., Linear Search, Binary Search). | Useful for searching databases, file systems, and retrieving information from large datasets. |
Graph Algorithms | Manage and traverse through graphs (e.g., Dijkstra’s Algorithm, DFS, BFS). | Enable pathfinding in maps, social network analysis, and traffic optimization. |
Dynamic Programming | Break problems into smaller subproblems and store results (e.g., Fibonacci Series, Knapsack Problem). | Applied in resource allocation, route planning, and financial modeling. |
Why You Should Learn Data Structures and Algorithms
Mastering data structures and algorithms is more than just a technical requirement—it’s a crucial skill that can transform how you approach coding problems. Here’s why:
- Enhanced Problem-Solving Skills: Understanding these concepts allows you to break down complex problems into manageable pieces and choose the most suitable solutions.
- Optimized Performance: With a solid grasp of data structures and algorithms, you can write code that is not only correct but also performs optimally.
- Career Advancement: Most technical interviews focus heavily on data structures and algorithms, making them essential for securing jobs at top tech companies.
Real-World Applications of Data Structures and Algorithms
- Search Engines: Google’s complex algorithms enable it to index millions of web pages and provide the most relevant results in milliseconds.
- Social Media Platforms: Data structures and algorithms determine what appears in your feed based on your interactions and preferences.
- Recommendation Systems: Netflix and Amazon use algorithms to suggest movies or products, enhancing user experience.
- Cryptography: Algorithms encrypt sensitive data, ensuring secure communication and data protection.
- Artificial Intelligence: Machine learning models rely heavily on complex algorithms to make decisions based on large datasets.
Conclusion
Understanding data structures and algorithms is like learning the language of problem-solving in programming. Whether you’re a beginner aiming to build a strong foundation or a professional seeking to enhance your skills, mastering these concepts will open up a world of possibilities. By combining the right data structures with efficient algorithms, you can solve real-world challenges more effectively and become a better programmer.
FAQs
Why are data structures and algorithms crucial for programming?
Data structures and algorithms are essential because they help organize data and define the steps to solve problems efficiently. Without them, even simple programs can become slow and resource-intensive.
How can I start learning data structures and algorithms?
Begin with basic data structures like arrays and linked lists, then move on to understanding algorithms such as sorting and searching. Practice solving problems on platforms like LeetCode or HackerRank.
Which is the best algorithm for searching?
For small datasets, Linear Search is straightforward. For larger, sorted datasets, Binary Search is more efficient as it reduces the search space by half at each step.
Can mastering data structures and algorithms help in interviews?
Absolutely! Most coding interviews focus on problem-solving using data structures and algorithms. Mastering them is essential for landing a job at top tech firms.
How are algorithms used in day-to-day applications?
Algorithms are behind almost every technology you use—from navigating GPS routes to predicting your next favorite TV show.