Table of Contents
When learning to program in Python, understanding how to work with variables and data types in Python is essential. These concepts form the foundation for creating and managing data in any software application. Variables store information that can be used and modified throughout a program, while data types define the kind of data a variable holds, such as numbers, text, or lists. In this blog, we’ll explore what variables are, how to take user input, the most common data types, and their importance in Python programming. Whether you are a beginner or an experienced developer, mastering variables and data types in python ensures you can write efficient and error free code.
Why Variables and Data Types in Python is Important?
Knowing how to use variables and data types in python efficiently ensures your code is more readable and easier to maintain. The ability to handle multiple data types makes Python versatile, allowing you to build a variety of applications—from simple scripts to complex data science projects.
Data types play a crucial role in error prevention. For instance, Python will raise a TypeError
if you try to perform arithmetic on a string without converting it to a number.
x = "10"
y = 2
print(int(x) + y) # Output: 12
In the above example, x
is converted from a string to an integer before adding it to y
. Understanding these nuances helps avoid common programming mistakes. Let’s understand variables and data types in python one by one in followings paragraphs:
Variables in Python?
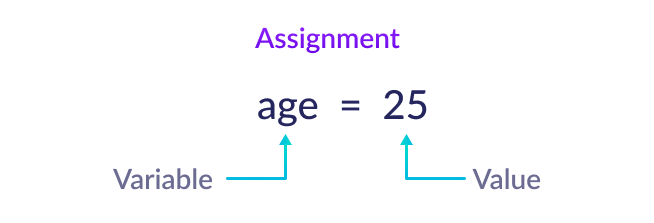
A variable is a named location in memory used to store data, which can change during the program’s execution. In Python, you don’t need to declare the type of a variable explicitly—it’s dynamically assigned based on the value stored. Let’s see an example:
name = "Alice"
age = 25
Here, name
holds a string, and age
holds an integer. Both variables can be reused or modified later in the code. This flexibility makes Python an excellent choice for beginners.
Rules for Defining Variables in Python
- Variable names must start with a letter or an underscore (
_
). For example:_name
orage
are valid, but1name
is not. - Variable names can contain letters, numbers, and underscores but cannot have spaces or special characters like
@
,!
, or%
. For example:user_name
is valid, butuser@name
is not. - Variable names are case-sensitive. For example:
Name
andname
are two different variables in Python. - Avoid using Python’s reserved keywords as variable names (e.g.,
if
,else
,while
). For example:class = 5
will result in a syntax error becauseclass
is a reserved keyword. - Use meaningful variable names to make your code easier to understand and maintain. For example:
user_age
is more descriptive than justx
. - Variables must be assigned a value before they are used. Let’s see an example:
name = "Alice" # Valid
print(name) # Output: Alice
print(age) # Error: 'age' is not defined
- Follow consistent naming conventions, such as snake_case for variables (e.g.,
first_name
) to enhance readability.
By following these rules, you can create well-structured code that is easy to read, understand, and maintain. Proper variable management is an essential skill as you explore more advanced variables and data types in Python.
Input in Python Using input()
The input()
function allows your program to receive user input during runtime. By default, the input is stored as a string, but it can be converted to other data types if needed. Let’s see an example on how to take input from user in Python:
name = input("Enter your name: ")
age = int(input("Enter your age: "))
print(f"Hello, {name}. You are {age} years old.")
In this code, the user provides their name and age. The age is converted to an integer using int()
, demonstrating how Python makes it easy to manage multiple data types within variables.
Data Types in Python
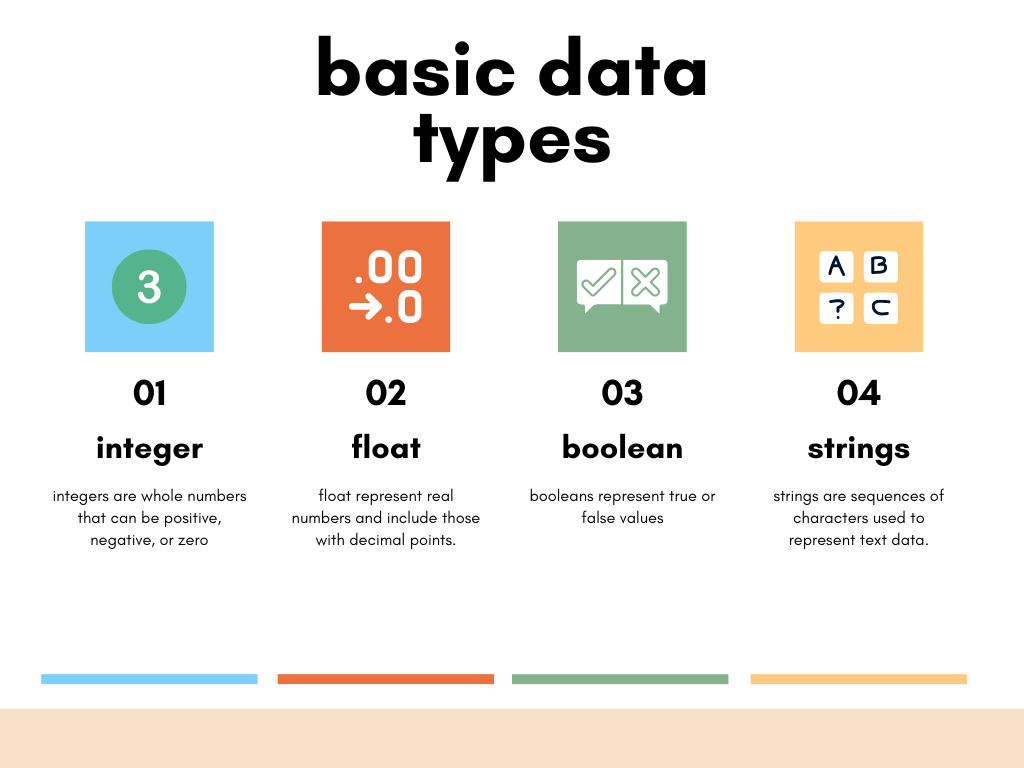
Python supports several data types, each with unique properties. Understanding variables and data types in Python allows you to manage and manipulate data effectively.
- Integers (
int
): Whole numbers, both positive and negative. Example:x = 42
- Floats (
float
): Numbers with decimal points. Example:pi = 3.14159
- Strings (
str
): Sequences of characters enclosed in single or double quotes. Example:greeting = "Hello, World!"
- Booleans (
bool
): Represent truth values:True
orFalse
. Example:is_active = True
- Lists (
list
): Ordered collections that can store multiple values. Example:fruits = ["apple", "banana", "cherry"]
Python’s flexibility allows you to switch between these data types dynamically, as needed. For example, you can use str()
, int()
, or float()
to convert one data type to another.
Conclusion
Mastering variables and data types in Python is the first step towards writing effective programs. Variables store data that can be used and modified, while data types define how the stored data behaves. Python’s dynamic typing simplifies development by eliminating the need for explicit declarations, making it a preferred language for beginners and professionals alike. As you continue to explore Python, you’ll find that a deep understanding of variables and data types is essential for developing everything from simple programs to complex machine learning models.
FAQs
What is a variable in Python?
A variable in Python is a named memory location that stores data, which can change during the program’s execution.
How do I take user input in Python?
Use the input()
function to accept user input. You can also convert the input to other data types using functions like int()
or float()
.
What are the common data types in Python?
Common data types include int
(integer), float
(decimal numbers), str
(strings), bool
(booleans), list
(collections), and tuple
(immutable collections).
Can Python variables store different data types?
Yes, Python variables are dynamically typed, meaning they can store different data types, and the type can change during runtime.
How do I change the data type of a variable in Python?
Use built-in functions like int()
, float()
, or str()
to convert between data types.
Why is understanding variables and data types in python important?
Understanding variables and data types in python helps in avoiding errors and ensures the correct operations are performed on variables.